Styled Components In React
- pankajsharmaaji1
- Sep 7, 2024
- 3 min read
Introduction
Styled Components is a powerful library for managing CSS in React applications, leveraging the CSS-in-JS approach to streamline styling. React, a popular JavaScript library for building user interfaces, enables developers to create interactive and reusable UI components. Styled Components integrates seamlessly with React, allowing developers to define and apply component-specific styles directly within their JavaScript code, enhancing both development efficiency and style management. Many React JS Interview Questions include question on Styled Components, thereby, making them integral for React learners.
All About Styled Components In React
Styled Components leverages tagged template literals to define styles. This means you can create styled elements by embedding CSS code within your JavaScript. The library generates unique class names for your components, ensuring that styles are applied only to the intended elements. This avoids the common issue of CSS conflicts and specificity problems.
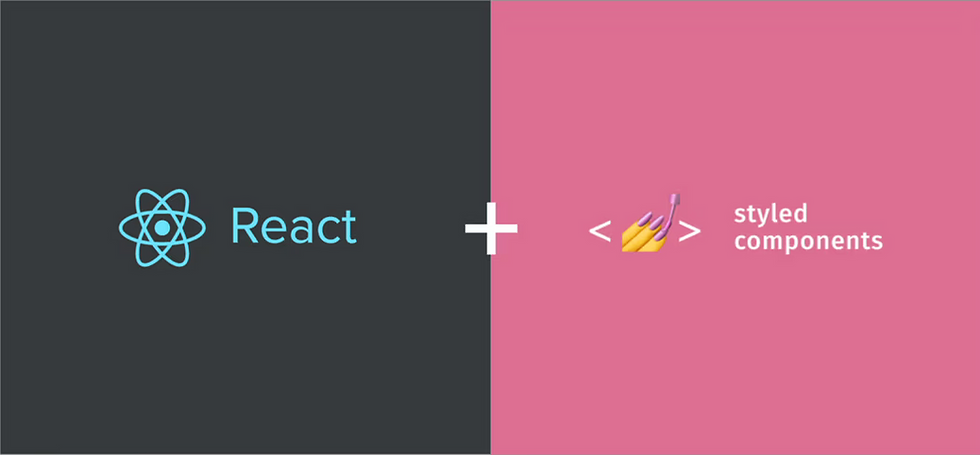
How to Use Styled Components?
Installation: To get started, you need to install Styled Components using npm or yarn:
“npm install styled-components”
or
“yarn add styled-components”
Basic Usage: Import the styled object from the library and use it to create styled components. Here’s an example:
“import styled from 'styled-components';
const Button = styled.button`
background-color: blue;
color: white;
padding: 10px 20px;
border: none;
border-radius: 5px;
font-size: 16px;
cursor: pointer;
&:hover {
background-color: darkblue;
}
`;
const App = () => {
return (
<div>
<Button>Click Me</Button>
</div>
);
};
export default App;”
In this example, Button is a styled component with defined styles. When you use <Button /> in your JSX, it applies the CSS specified within the template literal.
Props and Dynamic Styles: Styled Components also allows you to pass props to dynamically adjust styles. For instance:
“const Button = styled.button`
background-color: ${props => props.primary ? 'blue' : 'gray'};
color: white;
padding: 10px 20px;
border: none;
border-radius: 5px;
font-size: 16px;
cursor: pointer;
&:hover {
background-color: ${props => props.primary ? 'darkblue' : 'darkgray'};
}
`;
const App = () => {
return (
<div>
<Button primary>Primary Button</Button>
<Button>Secondary Button</Button>
</div>
);
};
export default App;”
Here, the primary prop controls the button's background color.
Theming: Styled Components supports theming through the ThemeProvider. This allows you to define global styles and variables:
“import { ThemeProvider } from 'styled-components';
const theme = {
primaryColor: 'blue',
secondaryColor: 'gray',
};
const Button = styled.button`
background-color: ${props => props.theme.primaryColor};
color: white;
padding: 10px 20px;
border: none;
border-radius: 5px;
font-size: 16px;
cursor: pointer;
&:hover {
background-color: ${props => props.theme.primaryColor};
}
`;
const App = () => {
return (
<ThemeProvider theme={theme}>
<div>
<Button>Styled Button</Button>
</div>
</ThemeProvider>
);
};
export default App;”
Advantages
Scoped Styles: Avoids CSS conflicts by scoping styles to components.
Dynamic Styling: Easily handle dynamic styles based on props or themes.
Readability: Keeps styles close to the component logic, improving readability.
Thus, Styled Components provides a powerful, modern way to manage styles in React applications, enhancing both development experience and maintainability. Refer to the React Course for Beginners to learn more about React and its various components.
Conclusion
Styled Components offers a streamlined, scalable approach to styling React applications by integrating CSS directly within JavaScript. With scoped styles, dynamic theming, and enhanced readability, it simplifies styling management and avoids common CSS issues, making it a valuable tool for modern React development.
Comments