What Are The Events In React?
- pankajsharmaaji1
- Jun 24, 2024
- 3 min read
Introduction
Events in React are fundamental to creating interactive and dynamic web applications. They capture user interactions like clicks, key presses, and form submissions, and allow developers to respond to these actions. React enhances event handling through Synthetic Events, which ensure cross-browser compatibility and improve performance. The React Certification Course provides the best training for aspiring professionals.
Understanding how to effectively manage these events is essential for building responsive and efficient applications using React's powerful component-based architecture.
All About Events In React
Events in React are mechanisms through which user interactions with a web application are captured and handled. They are analogous to events in standard HTML and JavaScript but come with their own React-specific enhancements and considerations. Understanding events in React is crucial for creating dynamic and responsive applications.
This comprehensive overview explores the nature of events in React, their handling, and best practices.
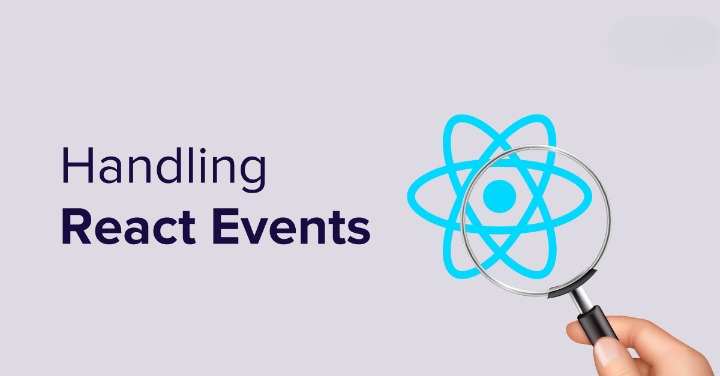
Nature of Events in React
Synthetic Events
React uses a concept called Synthetic Events. These are objects that wrap around the native browser events, providing a consistent interface across different browsers. Synthetic events are implemented to mimic the behavior of native events and are used to ensure compatibility and performance across different environments.
Event Pooling
One key feature of synthetic events is event pooling. React reuses event objects to improve performance. This means that the properties of the event object will be nullified after the event handler has been called. To use the event asynchronously, you must call event.persist() to remove the event from the pool and retain its properties.
Common Event Types
React supports a wide array of events, categorized broadly into:
Mouse Events: Such as onClick, onDoubleClick, onMouseEnter, onMouseLeave.
Keyboard Events: Such as onKeyDown, onKeyPress, onKeyUp.
Form Events: Such as onChange, onSubmit, onInput.
Focus Events: Such as onFocus, onBlur.
Touch Events: Such as onTouchStart, onTouchMove, onTouchEnd.
UI Events: Such as onScroll.
Clipboard Events: Such as onCopy, onCut, onPaste.
Composition Events: Such as onCompositionStart, onCompositionUpdate, onCompositionEnd.
Media Events: Such as onLoadStart, onTimeUpdate, onEnded.
Event Handling in React
Handling events in React involves attaching event listeners to React components. The React JS Course with Placement trains aspiring professionals in this field. Here’s how you can handle different types of events:
Inline Event Handlers
Inline event handlers are defined directly in the JSX. For example:
“<button onClick={this.handleClick}>Click me</button>”
Event Handler Methods
Typically, event handlers are methods of a component class or functions within a functional component. For instance:
“class MyComponent extends React.Component {
handleClick = () => {
console.log('Button clicked');
};
render() {
return <button onClick={this.handleClick}>Click me</button>;
}
}”
Passing Arguments
Sometimes you need to pass additional arguments to event handlers. This can be done using arrow functions or the bind method:
“<button onClick={(e) => this.handleClick(id, e)}>Click me</button>”
or
“<button onClick={this.handleClick.bind(this, id)}>Click me</button>”
Functional Components and Hooks
With the introduction of hooks, functional components can now handle events similarly to class components. Consider joining the React Certification Course for the best guidance. The useState and useEffect hooks are often used in conjunction with event handlers:
“const MyComponent = () => {
const [count, setCount] = useState(0);
const handleClick = () => {
setCount(count + 1);
};
return <button onClick={handleClick}>Click me</button>;
};”
Best Practices
Avoid Binding in Render
Binding event handlers in the render method can lead to performance issues because it creates a new function on each render. Instead, bind them in the constructor or use class fields:
“constructor(props) {
super(props);
this.handleClick = this.handleClick.bind(this);
}”
or
“class MyComponent extends React.Component {
handleClick = () => {
// Handle click
};
}”
Prevent Default Behavior
To prevent the default behavior of an event, use event.preventDefault() within the handler:
“handleSubmit = (event) => {
event.preventDefault();
// Handle form submission
};”
Event Delegation
React’s synthetic events handle event delegation efficiently at the root of the component tree, so you don’t need to manually delegate events as you might in vanilla JavaScript.
Conclusion
Events in React are a powerful and flexible way to handle user interactions. By leveraging synthetic events, React ensures cross-browser compatibility and performance. The React JS Course with Placement provides the best training and placement opportunities. Whether you’re working with class components or modern functional components with hooks, understanding and effectively handling events is fundamental to building interactive React applications. By following best practices, you can write clean, efficient, and maintainable event-handling code.
Comments