What Are DOM Events In React?
- pankajsharmaaji1
- Jul 29, 2024
- 3 min read
Introduction
DOM events are critical for creating interactive web applications, allowing users to interact with elements like buttons and forms. In React, a powerful JavaScript library for building user interfaces, DOM events are handled using a synthetic event system. This system provides a consistent, cross-browser interface, simplifying event handling for developers.
This blog explores React's approach to DOM events, its benefits, and best practices for efficient and responsive applications.
Everything You Need To Know About React DOM Events
DOM events are integral to building interactive web applications, and React, a popular JavaScript library for building user interfaces, offers a robust way to handle these events. DOM (Document Object Model) events are actions that occur in the browser, such as clicks, key presses, or mouse movements. Aspiring professionals can learn React Online to know more about the DOM Events. React provides a synthetic event system that wraps around the native DOM events, offering a consistent interface across different browsers.
Let us explore how DOM events are handled in React, the benefits of React's synthetic event system, and best practices for using events in React applications.
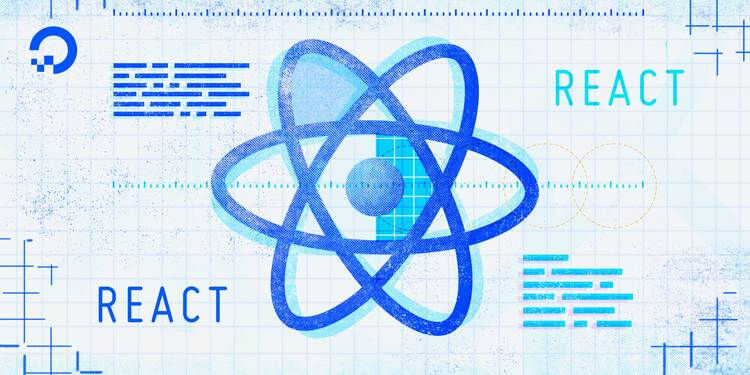
Understanding React's Synthetic Events
React's synthetic events are a cross-browser wrapper around the browser's native event system. This means that React handles event normalization, ensuring that the events behave consistently across all browsers. When an event occurs, React creates a synthetic event object that mimics the native event object, but with some enhancements and standardization. This synthetic event system is lightweight and provides an interface similar to the native event system, making it easy for developers to use.
Commonly Used DOM Events in React
1. Mouse Events: These include events like onClick, onDoubleClick, onMouseEnter, onMouseLeave, onMouseMove, onMouseDown, and onMouseUp.
“function handleClick() {
console.log('Button clicked');
}
return <button onClick={handleClick}>Click Me</button>;”
2. Keyboard Events: These include onKeyDown, onKeyPress, and onKeyUp.
“function handleKeyPress(event) {
if (event.key === 'Enter') {
console.log('Enter key pressed');
}
}
return <input onKeyPress={handleKeyPress} />;”
3. Form Events: These include onChange, onSubmit, and onFocus.
“function handleChange(event) {
console.log('Input value:', event.target.value);
}
return <input onChange={handleChange} />;”
4. Touch Events: These include onTouchStart, onTouchMove, onTouchEnd, and onTouchCancel.
“function handleTouchStart() {
console.log('Touch started');
}
return <div onTouchStart={handleTouchStart}>Touch Me</div>;”
Benefits of React's Synthetic Events
Cross-Browser Compatibility: React's synthetic events abstract away the inconsistencies between different browsers, providing a uniform API.
Performance Optimization: React batches updates and event handling, which can improve performance by reducing the number of re-renders.
Event Delegation: React employs a single event listener at the root of the document, reducing the number of event listeners and improving performance.
Memory Management: Synthetic events are automatically pooled and reused by React, which helps in reducing memory overhead.
Best Practices for Handling Events in React
1. Use Functional Components: With React's shift towards functional components and hooks, using functional components for event handling is recommended.
“function App() {
const handleClick = () => {
console.log('Button clicked');
};
return <button onClick={handleClick}>Click Me</button>;
}”
2. Avoid Inline Event Handlers: While it's possible to define event handlers inline, it's better to define them as functions to keep the code clean and improve performance.
“// Less preferable
return <button onClick={() => console.log('Button clicked')}>Click Me</button>;
// Preferable
function handleClick() {
console.log('Button clicked');
}
return <button onClick={handleClick}>Click Me</button>;”
3. Use Event Delegation Wisely: While React handles event delegation for you, understanding it can help you write more efficient code, especially when dealing with dynamically generated elements.
4. Clean Up Event Handlers: If you're adding event listeners directly to the DOM (outside of React's synthetic event system), ensure that you clean up these listeners to prevent memory leaks.
“useEffect(() => {
const handleResize = () => {
console.log('Window resized');
};
window.addEventListener('resize', handleResize);
return () => {
window.removeEventListener('resize', handleResize);
};
}, []);”
Conclusion
Handling DOM events in React is streamlined by the synthetic event system, which ensures cross-browser compatibility and performance optimizations. By following best practices such as using functional components, avoiding inline event handlers, understanding event delegation, and cleaning up event handlers, developers can create responsive and efficient React applications. Aspiring professionals are suggested to join the React Certification Course for the best guidance. React's approach to event handling simplifies the development process, making it easier to build interactive user interfaces.
Comments