What Is A React Router?
- pankajsharmaaji1
- Aug 17, 2024
- 3 min read
Introduction
React Router is an essential library for managing navigation in React applications. It simplifies the process of creating and handling different routes in a single-page application (SPA), enabling seamless navigation without page reloads. By using React Router, developers can define routes, manage nested routes, and create dynamic, responsive navigation experiences. This tool is fundamental for building modern, interactive web applications with React.
All About React Routers
React Router is an essential library for managing navigation in React applications. The React JS Course offers ample training sessions on React Routers for the best skill development of the aspiring professionals.
Let's break it down step-by-step in an easy and straightforward manner.
What is React Router?
React Router is a standard library system built on top of React to create and manage routes in your application. Think of routes as different paths or URLs your app can navigate to. For example, a blog might have routes for the homepage, individual blog posts, an about page, and a contact page.
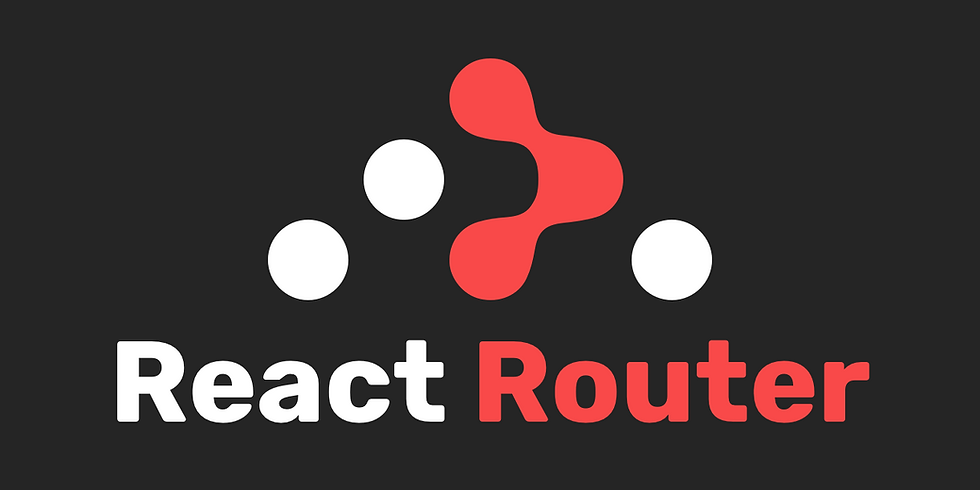
Why Use React Router?
When building single-page applications (SPAs) with React, you often want to display different content or pages based on the URL. Without React Router, managing these routes would be a complex task. React Router simplifies this by providing a robust solution to define and manage these routes seamlessly.
Key Concepts of React Router
1. Router
The Router is the main component that wraps your entire app. It listens to the URL changes in the browser and displays the corresponding components. There are different types of routers, like BrowserRouter and HashRouter.
BrowserRouter: Uses the HTML5 history API (pushState, replaceState, and popstate event) to keep your UI in sync with the URL.
HashRouter: Uses the hash portion of the URL (i.e., window.location.hash) to keep the UI in sync with the URL.
2. Route
A Route component is used to define a mapping between a URL path and the component that should be rendered. For instance, a route like /about would render the About component when the user navigates to www.yourwebsite.com/about.
“<Route path="/about" component={About} />”
3. Link
Link is a component used for navigation. Instead of using traditional anchor tags (<a>), which cause the page to reload, React Router's <Link> component allows for smooth, client-side navigation without refreshing the page.
“<Link to="/about">About</Link>”
4. Switch
Switch is a component that looks through all its children Route elements and renders the first one that matches the current URL. Consider checking the React JS Interview Questions for more information about React Routers. This is useful to render a 404 page when none of the routes match.
“<Switch>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
<Route component={NotFound} />
</Switch>”
5. Nested Routes
React Router supports nested routes, which means you can define routes within routes. This is particularly useful for layouts that have a common structure with specific sections that change based on the route.
“<Route path="/dashboard" component={Dashboard}>
<Route path="/dashboard/settings" component={Settings} />
</Route>”
Setting Up React Router
To use React Router in your application, you need to install it first. Refer to the React JS Course to learn how to set up React Router.
You can do this using npm or yarn:
“npm install react-router-dom
# or
yarn add react-router-dom”
Once installed, you can set it up in your main application file, typically App.js:
“import { BrowserRouter as Router, Route, Switch } from 'react-router-dom';
import Home from './Home';
import About from './About';
function App() {
return (
<Router>
<div>
<nav>
<Link to="/">Home</Link>
<Link to="/about">About</Link>
</nav>
<Switch>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
</Switch>
</div>
</Router>
);
}
export default App;”
Benefits of Using React Router
Declarative Routing: Define your routes in a declarative way, making the code more readable and easier to manage.
Dynamic Routing: React Router allows routes to be defined anywhere in the app, including in components that are dynamically loaded.
Nested Routing: Supports nested routes which enable complex layouts and UI structures.
Code Splitting: Integrates well with code splitting, allowing parts of your application to be loaded on demand, reducing the initial load time.
Active Links: Easily style links based on the current route using the NavLink component, which can apply active styles to the current link.
Conclusion
React Router is a powerful and flexible library that makes it easy to manage navigation in your React applications. It abstracts away the complexity of routing and provides a simple API to define routes, navigate between them, and manage the overall navigation flow of your app. The React JS Interview Questions include many questions on React Routers. Whether you're building a small website or a large web application, React Router is an invaluable tool to help you create a smooth and dynamic user experience.
Comments